Last week was about creating a simple program in scratch, so I took what I learned and created a simple game. It’s called protect the penguin, and you can play it here.
Building this simple game helped me understand abstraction and how helpful it can be when trying to create more complicated relationships. Instead of big blocks of code, I was able to create small descriptive functions that allowed me to focus on the high level functionality of the program. I also realized there are a multitude of ways to create the same game, and had to start over the first time because I felt like I had created a mess. I don’t think reaching the goal is the hardest part, it’s creating a system that is readable when you return to it after some length of time, making sure it isn’t difficult to modify if required at some point in the future.
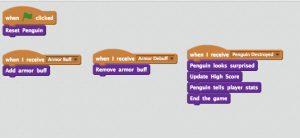
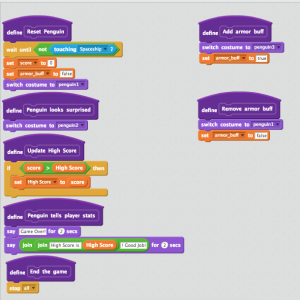
What is a function?
A function is a statement, or group of statements, grouped together to complete a specific task. In C main() is a function that runs the entire program. You can call your own functions from within main(), allowing the programmer to organize small tasks into functions with easy to understand function names, like “add_two_numbers.”
What are function arguments?
In the code above the add_two_numbers function has an “x” and “y” which are enclosed in parenthesis after the function name, and separated by a comma. When we define a function we can add as many arguments as we require to do some work inside of the definition. In this case we return the sum of our arguments.
Loops
When our program has to do a task many times, it makes sense to wrap those instructions within a loop. So far the class has discussed the for loop, and while loop.
While loops are good for running something until a condition is met. Maybe it is not necessary to know where you are in the repeated loop, you just keep going until some condition is true.
For loops offer a finer level of control due to the built in index variable required to create a for loop. This checks the state of “i” to see if it is less than or equal to 10. It’s important to realize the loop continues for as long as the expression is true. Also once evaluated to true we run the code within the parenthesis and then increment by the last instruction in the for loop. In this example I am incrementing by 1 but we could easily increment by any other value or decrement as well. Be careful of conditions that are never false, this will cause the loop to try to run forever, usually crashing the program.
Variables
A variable is a location in memory where data is stored under a label which is created by the programmer. In the physical world it is similar to a storage bin with a label you can write on with a marker.
In the example above I created three variables, an integer, a character, and an array of characters. Then I printed out a statement using those variables. On line 16 I change the number variable. Note I do not need to specify the variable type int anymore before the variable name, that is only needed when I create the variable for the first time. Also note my comment about the array. Once an array is created it can’t be modified. To change the string in my final statement I created a new char array called “new_string.”
Operators
Operators allow us to give logical or mathematical instructions in our programs. These are the operators available to us in C:
Arithmetic Operators
These operators are used for basic arithmetic.
Operators | Description |
+ | Addition or unary plus |
– | Subtraction or unary minus |
* | Multiplication |
/ | Division |
% | Modulo division |
- Variables used with operators are called operands.
- example: a * b
a and b are operands.
- Also note the ‘%’ cannot be used on the float data type
Relational Operators
Used when comparing values to one another.
Operators | Description |
< | Is less than |
<= | Is less than or equal to |
> | Is greater than |
>= | Is greater than or equal to |
== | Is equal to |
!= | Is not equal to |
You will find these operators used mostly within decision statements like if, and while loops.
Note that arithmetic operators have a higher priority than relational operators, therefore if arithmetic expressions exist on both sides of a relational expression, then both arithmetic expressions will be evaluated and then the relational expression will be evaluated.
example: ( 9 + 1 ) < ( 2 + 5 )
becomes: ( 10 ) < ( 7 )
finally: FALSE
Logical Operators
When several conditions in combination need to be tested or the inverse value needs to be tested.
Operators | Description |
&& | Logical AND |
|| | Logical OR |
! | Logical NOT |
When you combine two or more relational expressions you create a logical expression.
example: b > 15 || b < 30
The way precedence works in a little confusing because relational operators and logical operators weave in and out of priority. Here is the order from highest precedence (evaluates first) to lowest (evaluates last):
!,
, >=, <, <=, ==,
!=, &&, ||
So the Logical NOT operator happens before any relational operators, then all the relational operators come next, followed by the rest of the Logical operators.
Assignment Operators
Used to assign the result of an expression to a variable. There is the ‘=’ assignment operator as well as shorthand assignment operators. Shorthand assignment operators takes an expression like:
a = a + b;
and shortens it to:
a += b;
Operators | Description |
= | Assignment |
+= | Addition assignment |
-= | Subtraction assignment |
*= | Multiplication assignment |
/= | Division assignment |
%= | Modulo division assignment |
Increment and Decrement Operators
Increment and decrement operators are unary operators. These operators add 1 or subtract one from their operand. To be clear unary means the operator works on a single operand, unlike an operator that works on 2 operands.
example:
a + b (has 2 operands and an operator that evaluates to the sum of a and b)
a++ (has only one operand and evaluates to the sum of a + 1
Operators | Description |
++a | Increment (prefix operator) |
–a | Decrement (prefix operator) |
a++ | Multiplication (postfix operator) |
a– | Division (postfix operator) |
Note the difference between postfix and prefix operators is how they behave during variable assignment.
example:
a = 7; // a is given assignment to the value 7
z = 7; // z is given assignment to the value 7
b = a++; ( b is equal to a, which is 7, then a is incremented by 1 ).
c = ++z; // z is incremented first, then c is assigned the value of z which is 8).
finally b = 7 and c = 8.
Conditional Operators
There is only one operator here, but because the expression has either a true or false outcome, there is a pair of operators. The pair are known as ternary operators.
Operators | Description |
? : | exp1 ? true outcome : false outcome |
This allows us to take an if else statement and condense it down into one line. Whether this is better or worse for our program really depends. I can’t say I have enough experience at this point to make that distinction. I would however say at this point the ternary is “cleaner” looking on its own. I can imagine a big giant program might be harder to read though, without more readable lines of code like an if else block.
example:
an if else block:
if ( foo == bar )
baz = “matches”;
else
baz = “error”;
now with the ternary operator:
baz = (foo == bar) ? “matches” : “error”;
Bitwise Operators
In C, bitwise operators are used for shifting bits left or right. I really need to put more effort into understanding these operators, since they are used in memory efficient, embedded systems.
TO-DO: write an article about bitwise operators
Operators | Description |
& | Bitwise AND |
! | Bitwise OR |
^ | Bitwise exclusive OR |
<< | Bitwise Shift left |
>> | Bitwise Shift right |
~ | Bitwise Ones Complement |
example:
Special Operators
These operators unique behavior. They do not fit into any other category really. Also I really can’t say I know a ton about them at this point!
TO-DO: write an article about special operators
Operators | Description |
, | Comma Operator |
sizeof() | Sizeof Operator |
& | Pointer Address of Operator |
* | Value at operator (indirection operator) |
-> | Arrow Operator |
Wow. So that was a lot to write. I’ve been working on this blog post for way too long. There was a lot more to cover in this single lecture. I might be going too deep but I really want to cover as much as I can from the video lectures.
Just glad to have posted another article. Until next time.
I love your posts! I started in intense computer science program October. We meet 4 times per week, 12 hours total. We started with freecodecamp as a basis, them moved to MeanStack and used CodeSchool and Udemy. And all that with some class instruction as well, peer coding and many assignments and projects. We started CS50 in March. I am now on Week 3, Pset 4.
Struggling through it all lol. I so appreciate your CS50 posts. Great summaries, observations and hints. Thank you so much. I look forward to your next posts!
Hi Stephanie! Thank you for your kind words, I feel so motivated <3. Sometimes it's hard to get up so early before work and write these articles, and they take a lot of time. But knowing I am helping someone makes it worth it. Good luck with the rest of your classes. Very impressed with the courses you've taken so far!
Thanks Johnny! All the best to you and know that you are helping!
I can’t tell you how much this lesson overview has helped. The CS50 materials are not as clear as what I am reading here.
Thanks for the work you put into this. I hope it paid off for you in the end!
That is so kind of you to say <3 I'm really glad this helped you and i'll be posting content about what i'm up to very soon!